# Function Differences with torch.nn.init.uniform_ [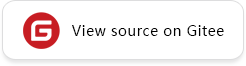](https://gitee.com/mindspore/docs/blob/r2.0/docs/mindspore/source_en/note/api_mapping/pytorch_diff/Uniform.md) ## torch.nn.init.uniform_ ```text torch.nn.init.uniform_( tensor, a=0.0, b=1.0 ) -> Tensor ``` For more information, see [torch.nn.init.uniform_](https://pytorch.org/docs/1.8.1/nn.init.html#torch.nn.init.uniform_). ## mindspore.ops.uniform ```text mindspore.ops.uniform(shape, minval, maxval, seed=None, dtype=mstype.float32) -> Tensor ``` For more information, see [mindspore.ops.uniform](https://www.mindspore.cn/docs/en/r2.0/api_python/ops/mindspore.ops.uniform.html). ## Differences PyTorch: The upper and lower bounds of uniform distribution are specified by parameters `a` and `b`, i.e. U(-a, b). MindSpore: The upper and lower bounds of uniform distribution are specified by parameters `minval` and `maxval`, i.e. U(minval, maxval),`seed` is used for random number generator to generate pseudorandom numbers. | Categories | Subcategories |PyTorch | MindSpore | Difference | | --- | --- | --- | --- |---| | Parameters | Parameter 1 | tensor | shape | The function is similar, but the input format is not.`tensor` is an n-dimensional tensor on Pytorch, but `shape` is a shape of random tensor to be generated on MindSpore platform.| | | Parameter 2 | a | minval | They have different names and similar functions, which are used to specify the minimum value of random values generated. | | | Parameter 3 | b | maxval | They have different names and similar functions, which are used to specify the maximum value of random values generated. | | | Parameter 4 | - | seed | Seed is used as entropy source for the random number engines to generate pseudo-random numbers, must be non-negative. | | | Parameter 5 | - | dtype | Specify the type of input data, and determine whether the data generated by uniform distribution is discrete or continuous according to the data type. | ## Code Example ```python # PyTorch import torch from torch import nn w = torch.empty(3, 2) output = nn.init.uniform_(w, a=1, b=4) print(tuple(output.shape)) # (3, 2) # MindSpore import numpy as np import mindspore from mindspore import ops from mindspore import Tensor shape = (3,2) minval = Tensor(1, mindspore.float32) maxval = Tensor(4, mindspore.float32) output = ops.uniform(shape, minval, maxval, dtype=mindspore.float32) print(output.shape) # Out: # (3, 2) ```